Best Mac Api Library For C++
Clients Libraries and Developer Tools Overview. RabbitMQ is officially supported on a number of operating systems and has several official client libraries. In addition, the RabbitMQ community has created numerous clients, adaptors and tools that we list here for your convenience.
C++Language | ||||
Standard Library Headers | ||||
Freestanding and hosted implementations | ||||
Named requirements | ||||
Language support library | ||||
Concepts library(C++20) | ||||
Diagnostics library | ||||
Utilities library | ||||
Strings library | ||||
Containers library | ||||
Iterators library | ||||
Ranges library(C++20) | ||||
Algorithms library | ||||
Numerics library | ||||
Input/output library | ||||
Localizations library | ||||
Regular expressions library(C++11) | ||||
Atomic operations library(C++11) | ||||
Thread support library(C++11) | ||||
Filesystem library(C++17) | ||||
Technical Specifications |
- Gtkmm is the official C interface for the popular GUI library GTK+.Highlights include typesafe callbacks, and a comprehensive set of widgets that are easily extensible via inheritance. You can create user interfaces either in code or with the Glade User Interface designer, using Gtk::Builder.There's extensive documentation, including API reference and a tutorial.
- Superpowered is the leading C Audio Library. Download it today. C audio libraries are critical for high performance audio programming since C is a language designed for.
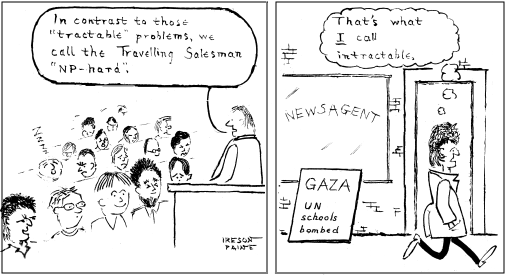
Language Support | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Concepts | |||||||||||||||||||||||||||||||||||||||||||||||||||
<concepts> | |||||||||||||||||||||||||||||||||||||||||||||||||||
Diagnostics | |||||||||||||||||||||||||||||||||||||||||||||||||||
General utilities | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Strings | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Localization | |||||||||||||||||||||||||||||||||||||||||||||||||||
Containers | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Iterators | |||||||||||||||||||||||||||||||||||||||||||||||||||
<iterator> | |||||||||||||||||||||||||||||||||||||||||||||||||||
Ranges | |||||||||||||||||||||||||||||||||||||||||||||||||||
<ranges> | |||||||||||||||||||||||||||||||||||||||||||||||||||
Algorithms | |||||||||||||||||||||||||||||||||||||||||||||||||||
Numerics | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Input/Output | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Regular expressions | |||||||||||||||||||||||||||||||||||||||||||||||||||
<regex> | |||||||||||||||||||||||||||||||||||||||||||||||||||
Filesystem support | |||||||||||||||||||||||||||||||||||||||||||||||||||
<filesystem> | |||||||||||||||||||||||||||||||||||||||||||||||||||
Thread support | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
C compatibility | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
The interface of C++ standard library is defined by the following collection of headers.
Creating a New System Photo LibraryThere are any number of reasons you might want to create a new system library in Photos. Maybe it’s become corrupted and Photos won’t open, or maybe you just want to start fresh and archive your old one.Regardless, to create a new System Photo Library, first open the location where your current system library is and drag it to a backup spot if you want to keep it (recommended). How do i move photos library to a new macbook pro.
Concepts library | ||
<concepts> (since C++20) | Fundamental library concepts | |
Coroutines library | ||
<coroutine> (since C++20) | Coroutine support library | |
Utilities library | ||
<cstdlib> | General purpose utilities: program control, dynamic memory allocation, random numbers, sort and search | |
<csignal> | Functions and macro constants for signal management | |
<csetjmp> | Macro (and function) that saves (and jumps) to an execution context | |
<cstdarg> | Handling of variable length argument lists | |
<typeinfo> | Runtime type information utilities | |
<typeindex> (since C++11) | std::type_index | |
<type_traits> (since C++11) | Compile-time type information | |
<bitset> | std::bitset class template | |
<functional> | Function objects, Function invocations, Bind operations and Reference wrappers | |
<utility> | Various utility components | |
<ctime> | C-style time/date utilites | |
<chrono> (since C++11) | C++ time utilites | |
<cstddef> | standard macros and typedefs | |
<initializer_list> (since C++11) | std::initializer_list class template | |
<tuple> (since C++11) | std::tuple class template | |
<any> (since C++17) | std::any class | |
<optional> (since C++17) | std::optional class template | |
<variant> (since C++17) | std::variant class template | |
<compare> (since C++20) | Three-way comparison operator support | |
<version> (since C++20) | supplies implementation-dependent library information | |
<source_location> (since C++20) | supplies means to obtain source code location | |
Dynamic memory management | ||
<new> | Low-level memory management utilities | |
<memory> | Higher level memory management utilities | |
<scoped_allocator> (since C++11) | Nested allocator class | |
<memory_resource> (since C++17) | Polymorphic allocators and memory resources | |
Numeric limits | ||
<climits> | limits of integral types | |
<cfloat> | limits of float types | |
<cstdint> (since C++11) | fixed-size types and limits of other types | |
<cinttypes> (since C++11) | formatting macros , intmax_t and uintmax_t math and conversions | |
<limits> | standardized way to query properties of arithmetic types | |
Error handling | ||
<exception> | Exception handling utilities | |
<stdexcept> | Standard exception objects | |
<cassert> | Conditionally compiled macro that compares its argument to zero | |
<system_error> (since C++11) | defines std::error_code , a platform-dependent error code | |
<cerrno> | Macro containing the last error number | |
Strings library | ||
<cctype> | Functions to determine the type contained in character data | |
<cwctype> | Functions to determine the type contained in wide character data | |
<cstring> | various narrow character string handling functions | |
<cwchar> | various wide and multibyte string handling functions | |
<cuchar> (since C++11) | C-style Unicode character conversion functions | |
<string> | std::basic_string class template | |
<string_view> (since C++17) | std::basic_string_view class template | |
<charconv> (since C++17) | std::to_chars and std::from_chars | |
<format> (since C++20) | Formatting library including std::format | |
Containers library | ||
<array> (since C++11) | std::array container | |
<vector> | std::vector container | |
<deque> | std::deque container | |
<list> | std::list container | |
<forward_list> (since C++11) | std::forward_list container | |
<set> | std::set and std::multiset associative containers | |
<map> | std::map and std::multimap associative containers | |
<unordered_set> (since C++11) | std::unordered_set and std::unordered_multiset unordered associative containers | |
<unordered_map> (since C++11) | std::unordered_map and std::unordered_multimap unordered associative containers | |
<stack> | std::stack container adaptor | |
<queue> | std::queue and std::priority_queue container adaptors | |
<span> (since C++20) | std::span view | |
Iterators library | ||
<iterator> | Range iterators | |
Ranges library | ||
<ranges> (since C++20) | Range access, primitives, requirements, utilities and adaptors | |
Algorithms library | ||
<algorithm> | Algorithms that operate on ranges | |
<execution> (since C++17) | Predefined execution policies for parallel versions of the algorithms | |
Numerics library | ||
<cmath> | Common mathematics functions | |
<complex> | Complex number type | |
<valarray> | Class for representing and manipulating arrays of values | |
<random> (since C++11) | Random number generators and distributions | |
<numeric> | Numeric operations on values in containers | |
<ratio> (since C++11) | Compile-time rational arithmetic | |
<cfenv> (since C++11) | Floating-point environment access functions | |
<bit> (since C++20) | Bit manipulation functions | |
<numbers> (since C++20) | Math constants | |
Input/output library | ||
<iosfwd> | forward declarations of all classes in the input/output library | |
<ios> | std::ios_base class, std::basic_ios class template and several typedefs | |
<istream> | std::basic_istream class template and several typedefs | |
<ostream> | std::basic_ostream, std::basic_iostream class templates and several typedefs | |
<iostream> | several standard stream objects | |
<fstream> | std::basic_fstream, std::basic_ifstream, std::basic_ofstream class templates and several typedefs | |
<sstream> | std::basic_stringstream, std::basic_istringstream, std::basic_ostringstream class templates and several typedefs | |
<syncstream> (since C++20) | std::basic_osyncstream, std::basic_syncbuf, and typedefs | |
<strstream> (deprecated in C++98) | std::strstream, std::istrstream, std::ostrstream | |
<iomanip> | Helper functions to control the format of input and output | |
<streambuf> | std::basic_streambuf class template | |
<cstdio> | C-style input-output functions | |
Localization library | ||
<locale> | Localization utilities | |
<clocale> | C localization utilities | |
<codecvt> (since C++11)(deprecated in C++17) | Unicode conversion facilities | |
Regular Expressions library | ||
<regex> (since C++11) | Classes, algorithms and iterators to support regular expression processing | |
Atomic Operations library | ||
<atomic> (since C++11) | Atomic operations library | |
Thread support library | ||
<thread> (since C++11) | std::thread class and supporting functions | |
<stop_token> (since C++20) | Stop tokens for std::jthread | |
<mutex> (since C++11) | mutual exclusion primitives | |
<shared_mutex> (since C++14) | shared mutual exclusion primitives | |
<future> (since C++11) | primitives for asynchronous computations | |
<condition_variable> (since C++11) | thread waiting conditions | |
<semaphore> (since C++20) | semaphores | |
<latch> (since C++20) | latches | |
<barrier> (since C++20) | barriers | |
Filesystem library | ||
<filesystem> (since C++17) | std::path class and supporting functions |
[edit]C compatibility headers
For some of the C standard library headers of the form xxx.h, the C++ standard library both includes an identically-named header and another header of the form cxxx (all meaningful cxxx headers are listed above).
Metal Api Mac
With the exception of complex.h , each xxx.h header included in the C++ standard library places in the global namespace each name that the corresponding cxxx header would have placed in the std namespace.
These headers are allowed to also declare the same names in the std namespace, and the corresponding cxxx headers are allowed to also declare the same names in the global namespace: including <cstdlib> definitely provides std::malloc and may also provide ::malloc. Including <stdlib.h> definitely provides ::malloc and may also provide std::malloc. This applies even to functions and function overloads that are not part of C standard library.
<assert.h> (deprecated) | behaves as if each name from <cassert> is placed in global namespace |
<ctype.h> (deprecated) | behaves as if each name from <cctype> is placed in global namespace |
<errno.h> (deprecated) | behaves as if each name from <cerrno> is placed in global namespace |
<fenv.h> (deprecated) | behaves as if each name from <cfenv> is placed in global namespace |
<float.h> (deprecated) | behaves as if each name from <cfloat> is placed in global namespace |
<inttypes.h> (deprecated) | behaves as if each name from <cinttypes> is placed in global namespace |
<limits.h> (deprecated) | behaves as if each name from <climits> is placed in global namespace |
<locale.h> (deprecated) | behaves as if each name from <clocale> is placed in global namespace |
<math.h> (deprecated) | behaves as if each name from <cmath> is placed in global namespace except for names of mathematical special functions |
<setjmp.h> (deprecated) | behaves as if each name from <csetjmp> is placed in global namespace |
<signal.h> (deprecated) | behaves as if each name from <csignal> is placed in global namespace |
<stdarg.h> (deprecated) | behaves as if each name from <cstdarg> is placed in global namespace |
<stddef.h> (deprecated) | behaves as if each name from <cstddef> is placed in global namespace except for names of std::byte and related functions |
<stdint.h> (deprecated) | behaves as if each name from <cstdint> is placed in global namespace |
<stdio.h> (deprecated) | behaves as if each name from <cstdio> is placed in global namespace |
<stdlib.h> (deprecated) | behaves as if each name from <cstdlib> is placed in global namespace |
<string.h> (deprecated) | behaves as if each name from <cstring> is placed in global namespace |
<time.h> (deprecated) | behaves as if each name from <ctime> is placed in global namespace |
<uchar.h> (deprecated) | behaves as if each name from <cuchar> is placed in global namespace |
<wchar.h> (deprecated) | behaves as if each name from <cwchar> is placed in global namespace |
<wctype.h> (deprecated) | behaves as if each name from <cwctype> is placed in global namespace |
[edit]Empty C headers
The headers <complex.h>
, <ccomplex>
, <tgmath.h>
, and <ctgmath>
do not contain any content from the C standard library and instead merely include other headers from the C++ standard library. The use of all these headers is deprecated in C++.
<ccomplex> (since C++11)(deprecated in C++17)(removed in C++20) | simply includes the header <complex> |
<complex.h> (deprecated) | simply includes the header <complex> |
<ctgmath> (since C++11)(deprecated in C++17)(removed in C++20) | simply includes the headers <complex> and <cmath>: the overloads equivalent to the contents of the C header tgmath.h are already provided by those headers |
<tgmath.h> (deprecated) | simply includes the headers <complex> and <cmath> |
[edit]Meaningless C headers
The headers <ciso646>
, <cstdalign>
, and <cstdbool>
are meaningless in C++ because the macros they provide in C are language keywords in C++.
<ciso646> (removed in C++20) | empty header. The macros that appear in iso646.h in C are keywords in C++ |
<iso646.h> (deprecated) | has no effect |
<cstdalign> (since C++11)(deprecated in C++17)(removed in C++20) | defines one compatibility macro constant |
<stdalign.h> (deprecated) | defines one compatibility macro constant |
<cstdbool> (since C++11)(deprecated in C++17)(removed in C++20) | defines one compatibility macro constant |
<stdbool.h> (deprecated) | defines one compatibility macro constant |
[edit]Unsupported C headers
The C headers <stdatomic.h>
, <stdnoreturn.h>
, and <threads.h>
are not included in C++ and have no cxxx equivalents.
[edit]Experimental libraries
C++ TR's/TS's also define several collections of headers.
[edit]See also
Language | ||||
Standard Library Headers | ||||
Freestanding and hosted implementations | ||||
Named requirements | ||||
Language support library | ||||
Concepts library(C++20) | ||||
Diagnostics library | ||||
Utilities library | ||||
Strings library | ||||
Containers library | ||||
Iterators library | ||||
Ranges library(C++20) | ||||
Algorithms library | ||||
Numerics library | ||||
Input/output library | ||||
Localizations library | ||||
Regular expressions library(C++11) | ||||
Atomic operations library(C++11) | ||||
Thread support library(C++11) | ||||
Filesystem library(C++17) | ||||
Technical Specifications |
Threads | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++11) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
this_thread namespace | |||||||||||||||||||||||||||||||||||||||||||||||||||
| |||||||||||||||||||||||||||||||||||||||||||||||||||
Mutual exclusion | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Generic lock management | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||
Condition variables | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++11) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++11) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++11) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++11) | |||||||||||||||||||||||||||||||||||||||||||||||||||
Semaphores | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20)(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
Latches and barriers | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
(C++20) | |||||||||||||||||||||||||||||||||||||||||||||||||||
Futures | |||||||||||||||||||||||||||||||||||||||||||||||||||
|
|
C++ includes built-in support for threads, mutual exclusion, condition variables, and futures.
|
[edit]Threads
Threads enable programs to execute across several processor cores.
Defined in header <thread> | |
(C++11) | manages a separate thread (class)[edit] |
(C++20) | std::thread with support for auto-joining and cancellation (class)[edit] |
Functions managing the current thread | |
(C++11) | suggests that the implementation reschedule execution of threads (function)[edit] |
(C++11) | returns the thread id of the current thread (function)[edit] |
(C++11) | stops the execution of the current thread for a specified time duration (function)[edit] |
(C++11) | stops the execution of the current thread until a specified time point (function)[edit] |
Thread cancellationThe
| (since C++20) |
[edit]Cache size access
min offset to avoid false sharing max offset to promote true sharing (constant)[edit] |
[edit]Mutual exclusion
Mutual exclusion algorithms prevent multiple threads from simultaneously accessing shared resources. This prevents data races and provides support for synchronization between threads.
Defined in header <mutex> | |
(C++11) | provides basic mutual exclusion facility (class)[edit] |
(C++11) | provides mutual exclusion facility which implements locking with a timeout (class)[edit] |
(C++11) | provides mutual exclusion facility which can be locked recursively by the same thread (class)[edit] |
(C++11) | provides mutual exclusion facility which can be locked recursively by the same thread and implements locking with a timeout (class)[edit] |
(C++17) | provides shared mutual exclusion facility (class)[edit] |
(C++14) | provides shared mutual exclusion facility and implements locking with a timeout (class)[edit] |
Generic mutex management | |
(C++11) | implements a strictly scope-based mutex ownership wrapper (class template)[edit] |
(C++17) | deadlock-avoiding RAII wrapper for multiple mutexes (class template)[edit] |
(C++11) | implements movable mutex ownership wrapper (class template)[edit] |
(C++14) | implements movable shared mutex ownership wrapper (class template)[edit] |
(C++11)(C++11)(C++11) | tag type used to specify locking strategy (class)[edit] |
(C++11)(C++11)(C++11) | tag constants used to specify locking strategy (constant)[edit] |
Generic locking algorithms | |
(C++11) | attempts to obtain ownership of mutexes via repeated calls to try_lock (function template)[edit] |
(C++11) | locks specified mutexes, blocks if any are unavailable (function template)[edit] |
Call once | |
(C++11) | helper object to ensure that call_once invokes the function only once (class)[edit] |
(C++11) | invokes a function only once even if called from multiple threads (function template)[edit] |
[edit]Condition variables
A condition variable is a synchronization primitive that allows multiple threads to communicate with each other. It allows some number of threads to wait (possibly with a timeout) for notification from another thread that they may proceed. A condition variable is always associated with a mutex.
(C++11) | provides a condition variable associated with a std::unique_lock (class) |
(C++11) | provides a condition variable associated with any lock type (class) |
(C++11) | schedules a call to notify_all to be invoked when this thread is completely finished (function) |
(C++11) | lists the possible results of timed waits on condition variables (enum) |
SemaphoresA semaphore is a lightweight synchronization primitive used to constrain concurrent access to a shared resource. When it's possible to use both, semaphore can be more efficient than condition variable.
Latches and BarriersLatches and barriers are thread coordination mechanism that allows any number of threads to block until an expected number of threads arrive at the barrier. Latches cannot be reused, barriers can be used repeatedly.
| (since C++20) |
[edit]Futures
The standard library provides facilities to obtain values that are returned and to catch exceptions that are thrown by asynchronous tasks (i.e. functions launched in separate threads). These values are communicated in a shared state, in which the asynchronous task may write its return value or store an exception, and which may be examined, waited for, and otherwise manipulated by other threads that hold instances of std::future or std::shared_future that reference that shared state.
(C++11) | stores a value for asynchronous retrieval (class template)[edit] |
(C++11) | packages a function to store its return value for asynchronous retrieval (class template)[edit] |
(C++11) | waits for a value that is set asynchronously (class template)[edit] |
(C++11) | waits for a value (possibly referenced by other futures) that is set asynchronously (class template)[edit] |
(C++11) | runs a function asynchronously (potentially in a new thread) and returns a std::future that will hold the result (function template)[edit] |
(C++11) | specifies the launch policy for std::async (enum)[edit] |
(C++11) | specifies the results of timed waits performed on std::future and std::shared_future (enum)[edit] |
Future errors | |
(C++11) | reports an error related to futures or promises (class)[edit] |
(C++11) | identifies the future error category (function)[edit] |
(C++11) | identifies the future error codes (enum)[edit] |